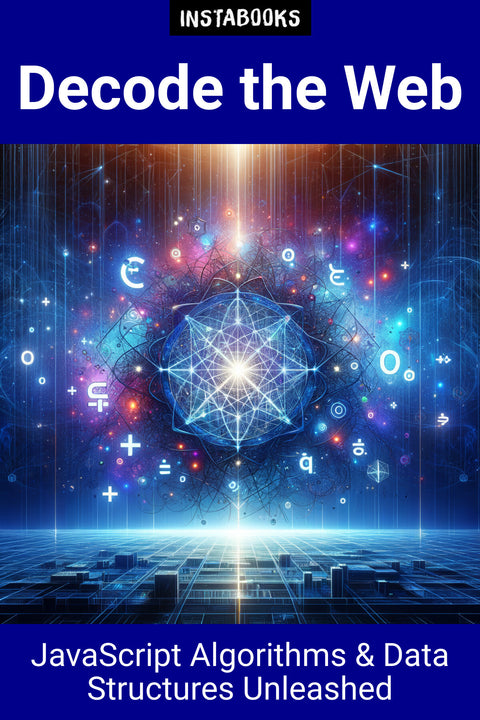
Decode the Web
JavaScript Algorithms & Data Structures Unleashed
AI Textbook - 100+ pages



Unlock the Foundations of JavaScript Mastery
"Decode the Web: JavaScript Algorithms & Data Structures Unleashed" offers a groundbreaking exploration into the core elements that underpin modern web development. This comprehensive guide dives deep into the essence of JavaScript - the world's most popular programming language - providing learners of every level with the tools to develop robust and efficient code.
Written by leading experts in the field, the content spans from fundamental concepts to advanced algorithmic strategies, ensuring a well-rounded education. The chapters are meticulously designed to gradually introduce readers to the complexities of data structures and algorithms, making even the most intricate topics accessible.
Whether you're a beginner eager to understand the basics or an expert looking to deepen your knowledge of advanced theories, this book is tailored to cater to a broad spectrum of readers. Practical examples and exercise are interspersed throughout the chapters, enabling hands-on learning and application of theoretical knowledge in real-world scenarios.
"Decode the Web" is not just a book; it's an invaluable resource that provides a unique perspective on solving common programming challenges using JavaScript. It presents a perfect blend of theory and practice, enriched with tips and strategies to enhance your coding skills.
Step into the world of JavaScript Algorithms and Data Structures with confidence. Elevate your coding expertise and prepare to transform your ideas into efficient, scalable applications with this masterclass in web development.
Table of Contents
1. Introduction to JavaScript- The Evolution of JavaScript
- Key Concepts and Syntax
- Setting Up Your Development Environment
2. Fundamentals of Data Structures
- Primitive Types and Operations
- Arrays and Objects
- Introduction to Hash Tables
3. Exploring Algorithms
- Understanding Algorithms
- Sorting Algorithms
- Searching Algorithms
4. Advanced Data Structures
- Stacks and Queues
- Linked Lists
- Trees and Graphs
5. Algorithm Analysis
- Time Complexity
- Space Complexity
- Big O Notation
6. Mastering Recursion
- The Concept of Recursion
- Recursive Functions in JavaScript
- Practical Recursive Problems
7. Dynamic Programming
- Introduction to Dynamic Programming
- Memoization and Tabulation
- Dynamic Programming in Practice
8. Dealing with Complexity
- Greedy Algorithms
- Divide and Conquer
- Backtracking Algorithms
9. Web Development with JavaScript
- The Document Object Model (DOM)
- Event Handling and Asynchronous Programming
- Building Responsive UIs
10. Testing and Debugging
- Writing Testable Code
- Unit Testing in JavaScript
- Debugging Techniques
11. Advanced Topics in JavaScript
- Closures and Scope
- Generators and Iterators
- Module Systems and Packaging
12. Final Projects
- Creating a Web Application
- Algorithm Challenges
- Data Structure Implementations